Keeping a running tally in MySQL
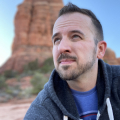
I have a database table in which I'm storing jobs for multiple companies. The job # needs to increment per company, which means I can't just use an auto-incrementing key. (Each company will have a job 1, 2, 3, 4, etc)
I somehow need to make sure it correctly increments +1 for each job assigned to the same company, even if multiple people are inputting jobs at the same time. Anyone know how can I accurately keep track of a running tally like that?
I somehow need to make sure it correctly increments +1 for each job assigned to the same company, even if multiple people are inputting jobs at the same time. Anyone know how can I accurately keep track of a running tally like that?
0
Comments
SELECT MAX(job_id)+1 FROM `job` WHERE `company` = 'x';
That will return the next ID for that company... you could also write a stored function.
Using a function like this will get you this:
It's a lot "cleaner" and makes it so you don't have to call 2 separate queries in your code.
1. You still execute two queries. One is just executed in the stored procedure.
2. This can simply be written as a sub-query.
For example...
INSERT INTO jobs COLS(..., job_id), VALS(..., (SELECT NextJobID=MAX(job_id)+1 FROM jobs WHERE company='%company%'))
This accomplishes the exact same thing without the need to maintain a stored procedure. Stored procedures are fine and dandy, but there is a trade-off between code maintainability and cleanliness.
As a general rule, stored procedures should only be used when they are actually functional in nature and will be used across multiple queries.
Since here you only have one use-case for this sub-query, abstracting it to a stored procedure introduces too much maintenance overhead to justify.
Also note that it is actually slower to call a stored procedure than it is to execute a trivial sub-query such as this. Function call overhead > trivial sub-query overhead.